Table of Contents
Cross-browser compatibility issues can be a real headache for web developers. Ensuring that your website looks and functions the same across different browsers is crucial for providing a seamless user experience. If you’ve encountered problems with your site not working as expected on various browsers, fret not! In this guide, we’ll walk you through some practical steps to fix cross-browser compatibility issues and make your website shine on every platform.
What Is a Cross-Browser Compatibility Issue?
A cross-browser compatibility issue refers to problems or inconsistencies that arise when a website or web application behaves differently on various web browsers. The term emphasizes the challenge of ensuring that a website functions properly and looks consistent across different browsers, such as Chrome, Firefox, Safari, Edge, and others.
Web developers need to consider that each browser may interpret HTML, CSS, and JavaScript code differently, leading to discrepancies in how a website is displayed or functions. Cross-browser compatibility issues can manifest in various ways, including layout inconsistencies, broken features, or design elements not rendering correctly.
These issues often arise due to differences in browser rendering engines, support for web standards, and the implementation of certain features. For example, a CSS property or JavaScript function that works seamlessly in one browser may not be supported or may behave differently in another.
Also read: CSS Grid Vs Flexbox – Features, Differences, Examples, and Codes
How to Identify Cross-Browser Compatibility Issues?
Identifying cross-browser compatibility issues is a crucial step in ensuring that your website functions consistently across different web browsers. Here are some practical methods to help you identify these issues:
Test Across Multiple Browsers
Use popular browsers such as Chrome, Firefox, Safari, Edge, and Internet Explorer to test your website. Each browser may interpret code differently, leading to variations in rendering and functionality.
Utilize Browser Developer Tools
Most modern browsers come equipped with developer tools. Use these tools to inspect elements, debug JavaScript, and analyze network requests. Pay attention to any error messages, warnings, or differences in the rendering of HTML and CSS.
Online Browser Testing Tools
Explore online tools that allow you to test your website on multiple browsers simultaneously. Platforms like BrowserStack, CrossBrowserTesting, or Sauce Labs provide virtual environments for testing across various browsers and versions.
Check Browser Compatibility Lists
Refer to browser compatibility lists or matrices provided by reputable sources such as MDN Web Docs or Can I Use. These resources highlight the support and compatibility of specific HTML, CSS, and JavaScript features across different browsers.
User Feedback
Encourage users to provide feedback on their experience with your website. Users on different browsers may encounter issues that you might not be aware of. Analyze user feedback and prioritize fixing issues that impact a significant portion of your audience.
Device Testing
Test your website on various devices, including desktops, laptops, tablets, and smartphones. Different devices may have different screen sizes and resolutions, leading to unique compatibility challenges.
Validate HTML and CSS Code
Use online validation tools to check the validity of your HTML and CSS code. Fixing syntax errors and adhering to web standards can often resolve compatibility issues.
Check Console Logs
Inspect the browser console logs for error messages, warnings, or deprecated features. Addressing issues reported in the console can help improve cross-browser compatibility.
Responsive Design Testing
Ensure that your website is responsive by testing it on devices with various screen sizes. This includes both desktop and mobile devices. Use media queries to apply specific styles based on screen dimensions.
Browser-Specific Testing
If you’re aware of specific browsers that your audience commonly uses, prioritize testing on those browsers. Addressing issues on widely used browsers first can have a significant impact on user satisfaction.
Also read: Add Toggle Up and Down Arrows With CSS Transform and Transition in a Menu
How to Fix Cross-Browser Compatibility Issues?
Cross-browser compatibility issues can be a headache for web developers due to the varying ways different browsers render web pages. However, with strategic approaches and leveraging CSS, these issues can be effectively addressed.
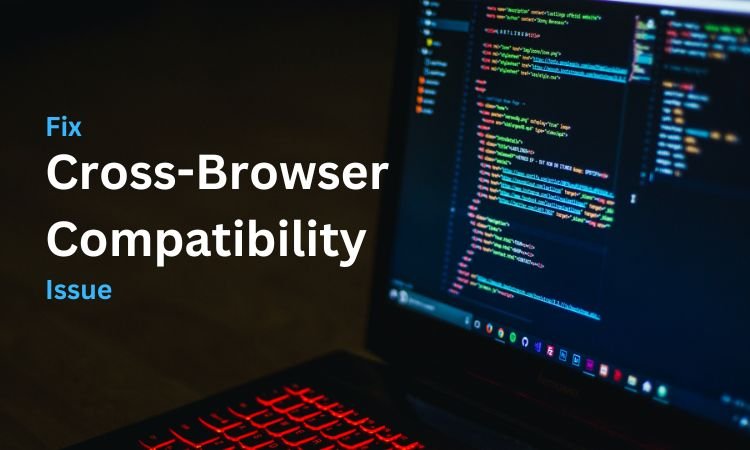
Let’s delve into how to fix cross-browser compatibility issues using CSS.
1. Normalize CSS
Use Normalize.css to establish consistent default styles across browsers.
<!-- Include Normalize.css in your project -->
<link rel="stylesheet" href="path/to/normalize.css" />
2. Use Vendor Prefixes
Apply vendor prefixes to CSS properties for consistent rendering.
/* Example with transform property */
.transformed-element {
-webkit-transform: rotate(45deg);
-moz-transform: rotate(45deg);
-ms-transform: rotate(45deg);
transform: rotate(45deg);
}
3. Test on Multiple Browsers
Utilize testing tools like BrowserStack or CrossBrowserTesting for thorough testing.
Manually test on different browsers during development.
4. Use CSS Resets
Apply CSS resets to ensure a consistent baseline for styling.
/* Example with Eric Meyer Reset CSS */
html, body, div, span, applet, object, iframe,
h1, h2, h3, h4, h5, h6, p, blockquote, pre,
a, abbr, acronym, address, big, cite, code,
del, dfn, em, img, ins, kbd, q, s, samp,
small, strike, strong, sub, sup, tt, var,
b, u, i, center,
dl, dt, dd, ol, ul, li,
fieldset, form, label, legend,
table, caption, tbody, tfoot, thead, tr, th, td,
article, aside, canvas, details, embed,
figure, figcaption, footer, header, hgroup,
menu, nav, output, ruby, section, summary,
time, mark, audio, video {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
}
5. Avoid Browser-Specific Hacks
Minimize the use of browser-specific hacks to maintain a clean codebase.
/* Example of a browser-specific hack targeting Internet Explorer */
.ie-specific-element {
/* Styles for other browsers */
background-color: blue;
/* Styles specifically for Internet Explorer */
*background-color: red;
}
6. Use Feature Detection
Implement feature detection using JavaScript to apply styles selectively.
// Example using Modernizr library
if (Modernizr.flexbox) {
// Apply styles for browsers supporting Flexbox
} else {
// Apply alternative styles for browsers without Flexbox support
}
7. Use CSS Frameworks
Leverage CSS frameworks like Bootstrap for consistent styling.
<!-- Include Bootstrap CSS in your project -->
<link rel="stylesheet" href="path/to/bootstrap.css" />
Also read: Troubleshooting Common CSS Issues in WordPress
Tips to Avoid Cross-Browser Compatibility Issues
Ensuring your website works seamlessly across different browsers is crucial for providing a consistent user experience. To avoid cross-browser compatibility issues in the production environment, consider the following 10 tips:
1. Validate HTML and CSS
- Different browsers interpret code differently. Validate your HTML and CSS to catch syntax errors early on.
- Write well-aligned code, insert comments where needed, and maintain proper indentation.
- Utilize validation tools like W3C HTML validator, Jigsaw CSS validator, and JS Lint for error-free code.
2. Maintain Layout Compatibility
- Create a responsive design that works on all devices and browsers.
- Use HTML viewport metatag to ensure content spans correctly on mobile screens.
- Implement CSS Multi-Column layouts, Flexbox, and Grid for maintaining proper content layout.
3. Use CSS Resets
- Override default browser styles by using CSS reset style sheets.
- Examples of reset style sheets include Normalize.css, HTML5 Reset, and Eric Meyer’s Reset CSS.
4. Provide Support for Basic Features
- Cross-check the application to ensure native features are supported across different browsers.
- Implement feature detection to provide alternative code for browsers that don’t support specific features.
- Consider using cross-browser automation tools like Testsigma for efficient testing.
5. Check JavaScript Issues
- Use JavaScript libraries that support a wide range of browsers and features.
- Transpile JavaScript code using the latest features of ES6/ECMAScript for compatibility with older browsers.
6. Check DOCTYPE Tag
- Define the DOCTYPE tag in your code to inform browsers about the rules to follow.
- Missing DOCTYPE tags may lead to browsers entering quirk mode, causing non-standard behavior.
7. Test on Real Devices
- Test your application on real desktops, mobiles, tablets, and laptops to identify issues early.
- Consider using cross-browser testing tools like Testsigma for immediate access to a variety of browser-OS combinations.
8. Use Frameworks and Libraries
- Opt for frameworks and libraries that support cross-browser compatibility.
- For JavaScript, consider using jQuery, AngularJS, or ReactJS. For CSS, Bootstrap, Foundation, and 960 grid are reliable choices.
9. Cross-Browser Test Early
- Start cross-browser testing as soon as one page of the application is ready.
- Early testing helps uncover compatibility issues in the development cycle, allowing for quick fixes.
- Minimize stress and challenges later in the cycle by addressing compatibility concerns early on.
10. Keep Frameworks and Libraries Updated
- Regularly update the frameworks and libraries you use in your web development projects.
- Frameworks and libraries often release updates to improve compatibility with new browser versions and fix known issues.
- Staying current ensures that your website benefits from the latest enhancements and remains compatible with evolving browser technologies.
Also read: How to Fix Common HTML Validation Errors on Your Website?
Final Thought
Fixing cross-browser compatibility issues may seem challenging, but with attention to detail and a systematic approach, you can ensure that your website performs seamlessly across different browsers. By following these practical tips, you’ll be well on your way to providing a consistent and enjoyable user experience for visitors using any browser.